yarn berry를 사용하여 typescript 개발 환경을 만들어보자
1. yarn berry 설정
패키지 매니저 버전을 yarn berry로 변경하기 위해, 아래 명령어를 수행한다.
<shell />yarn set version berry
위 명령어가 수행이 되면, .yarn 폴더, .yarnrc.yml 파일이 생성된다.
2. 프로젝트 생성
yarn init 명령어를 사용하여, 프로젝트를 생성한다.
<shell />yarn init -y
package.json을 보면 packageManager 부분이 yarn@3.x.x과 설정되어 있는 것을 확인 할 수 있다. (3버전이 berry)
<javascript />
{
...
"packageManager": "yarn@3.3.1",
...
}
3. typescript 설정
기본적으로 프로젝트가 설정되었으니 typescript를 설정해보자. typescript를 사용하기 위해서는 아래 의존성이 필요하다.
- typescript: typescript를 사용하기 위한 코어 라이브러리
- @types/node: nodejs(https://nodejs.org/en/)에서 사용하는 built-in module의 타입을 정의해놓은 라이브러리
<shell />yarn add --dev typescript @types/node
typescript가 설치되었으면, tsc 명령어를 사용할 수 있다.
tsc 명령어를 사용하여, tsconfig.json을 생성해보자. (의존성을 global로 설치한경우, 바로 tsc로 시작하여 커맨드를 사용할 수 있지만, 본 글에서는 dev dependency로 설치하였기 때문에 앞에 yarn을 붙여야 함)
<shell />yarn tsc --init
tsconfig.json 파일이 생성된 것을 확인 할 수 있는데, 기본 설정에서 아래 부분만 주석을 해제하였다. 그리고, outDir을 dist로 변경했다.
주석에도 설명이 있지만, 간단히 설명하면
- declaration: d.ts 파일을 생성해줘서 프로젝트의 코드의 타입을 모두 정의해 줌 (declarationMap 옵션을 사용하려면 true로 설정해야함)
- declarationMap: 디버깅을 위해 원본 소스와 컴파일된 javascript 코드를 맵핑해 줌
- outDir: typescript 파일을 javascript로 컴파일하고 결과 산출물을 생성하는 위치를 뜻함
- removeComments: 원본 코드의 주석을 모두 제거
<shell />{ "compilerOptions": { ... "declaration": true, /* Generate .d.ts files from TypeScript and JavaScript files in your project. */ "declarationMap": true, /* Create sourcemaps for d.ts files. */ // "emitDeclarationOnly": true, /* Only output d.ts files and not JavaScript files. */ // "sourceMap": true, /* Create source map files for emitted JavaScript files. */ // "outFile": "./", /* Specify a file that bundles all outputs into one JavaScript file. If 'declaration' is true, also designates a file that bundles all .d.ts output. */ "outDir": "./dist", /* Specify an output folder for all emitted files. */ "removeComments": true, /* Disable emitting comments. */ ... } }
그리고, compilerOptions 아래에 include, exclude도 정의한다.
<typescript />
{
...
"include": ["src/**/*.ts"],
"exclude": ["node_modules", "dist", "**/*.(test|spec).ts"]
}
4. 테스트 코드 작성
너무 간단하게도 typescript 설정이 끝났다. 이제 간단하게 테스트해 볼 코드를 작성해보자
src/index.ts 파일을 생성한다. 그리고, 코드를 작성한다. ("인사" 를 하는 메소드를 생성하고 호출하는 코드)
<typescript />
const greet = (name: string) => console.log(`hello, ${name}`);
const names = ['bluemiv', 'javascript', 'typescript'];
names.forEach(greet);
5. typescript 빌드
아래 명령어를 사용하여, 컴파일을 수행해보자. (tsconfig.json 에 컴파일 설정을 모두 해줬기 때문에 따로 옵션을 추가할 필요 없음)
<shell />yarn tsc
컴파일이 끝나면, 프로젝트 내에 dist 디렉토리가 생성이 된다.
- index.d.ts: declaration 옵션에 의해 타입 정의 파일이 생성됨
- index.d.ts.map: declarationMap 옵션에 의해 원본 소스와 javascript 코드의 맵핑 정보가 담긴 파일이 생성됨
- index.js: javascript로 컴파일된 파일
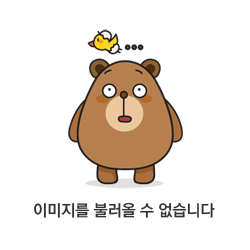


javascript로 변환됐으니 실행해보면
<shell />node dist/index.js
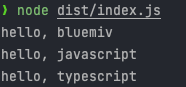
'Frontend > Typescript' 카테고리의 다른 글
Typescript 기본 타입(number, string, boolean, any, symbol ...) (0) | 2022.05.11 |
---|---|
Typescript란? 타입스크립트 기본 설정(컴파일 옵션, tsconfig.json) (0) | 2022.05.10 |